PyQt is a Python library for creating GUI applications using the Qt toolkit. Created by Riverbank Computing, PyQt is free software (GPL licensed) and has been in development since 1999. PyQt5 was released in 2016 and last updated in October 2021.
This complete PyQt5 tutorial takes you from first concepts to building fully-functional GUI applications in Python. It requires some basic Python knowledge, but no previous familiarity with GUI concepts. Everything will be introduced step by by step, using hands-on examples.
PyQt5 is the Qt5-based edition of the Python GUI library PyQt from Riverbank Computing.
There are two major versions currently in use: PyQt5 based on Qt5 and PyQt6 based on Qt6. Both versions are almost completely compatible aside from imports. PyQt6 also makes some changes to how namespaces and flags work, but these are easily manageable.
Looking for something else? I also have a PyQt6 tutorial, PySide2 tutorial and PySide6 tutorial.
This track consists of 36 tutorials. Keep checking back as I'm adding new tutorials regularly — last updated .
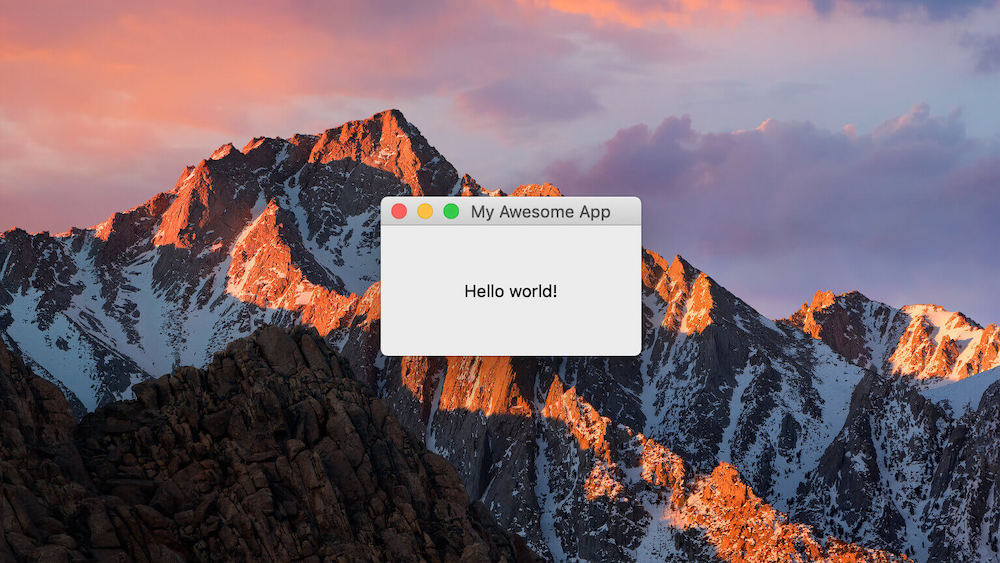
Getting started with PyQt5
Take your first steps building apps with Python & Qt5
Like writing any code, building PyQt5 applications is all about approaching it in the right way. In the first part of the course we cover the fundamentals necessary to get you building Python GUIs as quickly as possible. By the end of the first part you'll have a running QApplication
which we can then customize.
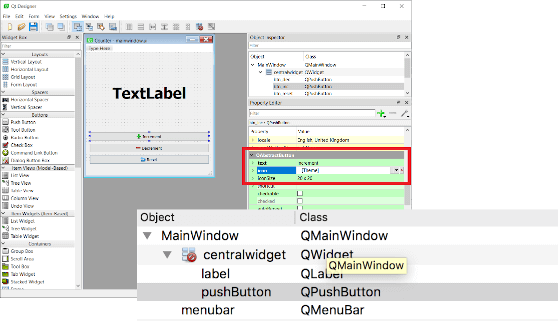
Creating applications with Qt Designer and PyQt5
Using the drag-drop designer to develop your apps
As your applications get larger or interfaces become more complicated, it can get a bit cumbersome to define all elements programmatically. The good news is that Qt comes with a graphical editor Qt Designer (or Qt Creator) which contains a drag-and-drop UI editor — Qt Designer. In this PyQt5 tutorial we'll cover the basics of creating Python GUIs with Qt Designer.
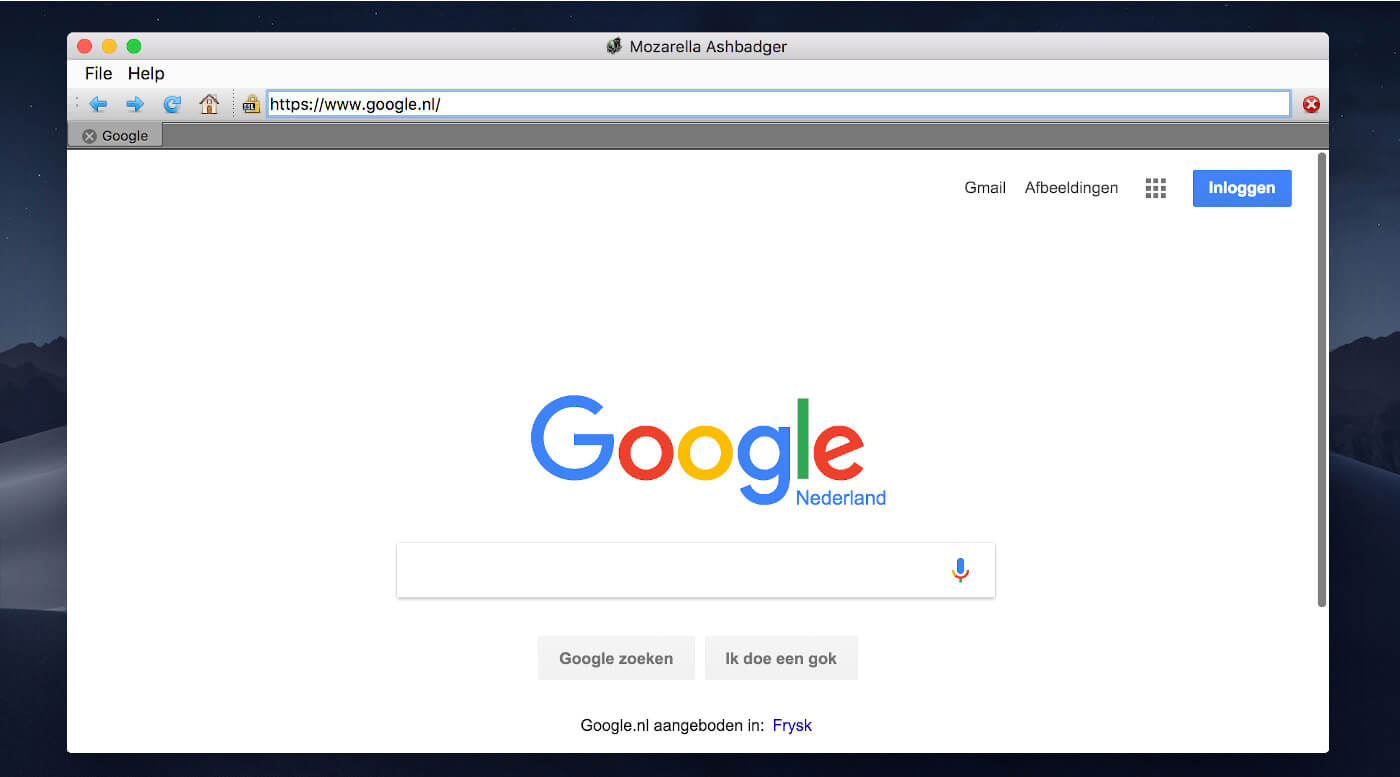
Build a Web Browser with PyQt5
Build your own tabbed web browser with PyQt5
Now we've learnt the basics, we'll put it into practice building a real-life app. In this course we'll create a functional web browser using Qt5 widgets. Starting with the basics and then gradually extending it to add features like opening and saving pages, help, printing and tabbed browsing. Follow the tutorial step by step to create your own app, but feel free to experiment as you go.
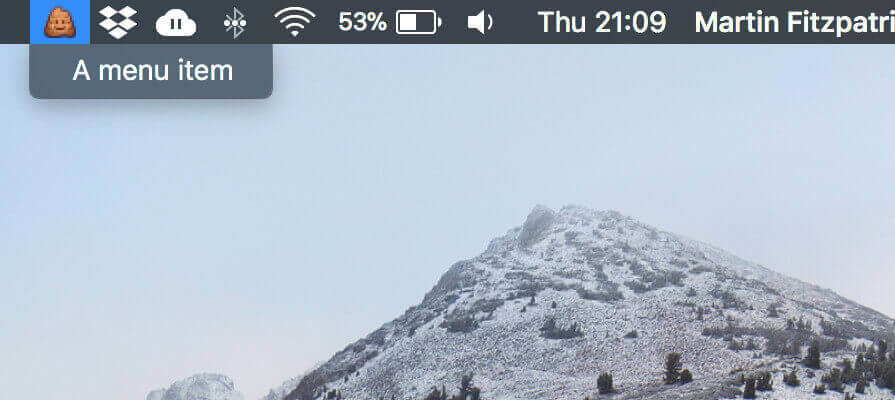
Extended UI features with PyQt5
Extending your apps with complex GUI behaviour
In this PyQt5 tutorial we'll cover some advanced features of Qt that you can use to improve your Python GUIs.
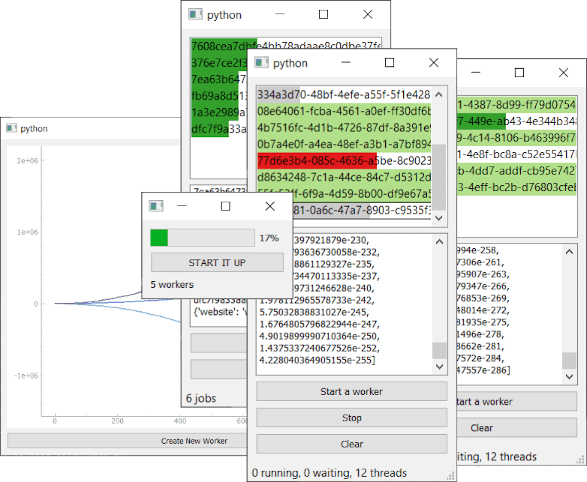
Threads & Processes in PyQt5
Run concurrent tasks without impacting your UI
As your applications become more complex you may finding yourself wanting to perform long-running tasks, such as interacting with remote APIs or performing complex calculations. By default any code you write exists in the same thread and process, meaning your long-running code can actually block Qt execution and cause your Python GUI app to "hang". In this PyQt5 tutorial we'll cover how to avoid this happening and keep your applications running smoothly, no matter the workload.
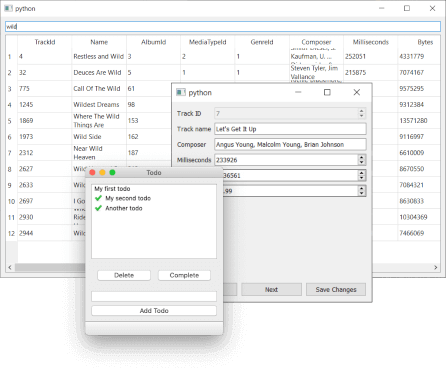
PyQt5 ModelViews and Databases
Connecting your application to data sources
All but the simplest of apps will usually need to interact with some kind of external data store — whether that's a database, a remote API or simple configuration data. The Qt ModelView architecture simplifies the linking and updating your UI with data in custom formats or from external sources. In this PyQt5 tutorial we'll discover how you can use Qt ModelViews to build high performance Python GUIs.
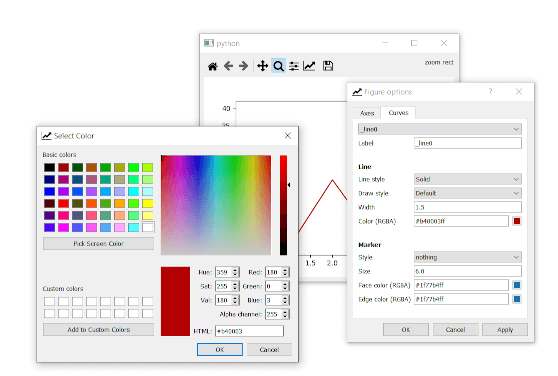
Graphics and Plotting in PyQt5
Vector graphics and plotting using PyQtGraph
Python is one of the most popular languages in the data science and machine learning fields. Effective visualization of data is a key part of building usable interfaces for data science. Matplotlib is the most popular plotting library in Python, and comes with support for PyQt built in. In addition, there are PyQt-specific plotting options available such as PyQtGraph which provide a better interactive experience. In this tutorial we'll look at these alternatives and build some simple plot interfaces.
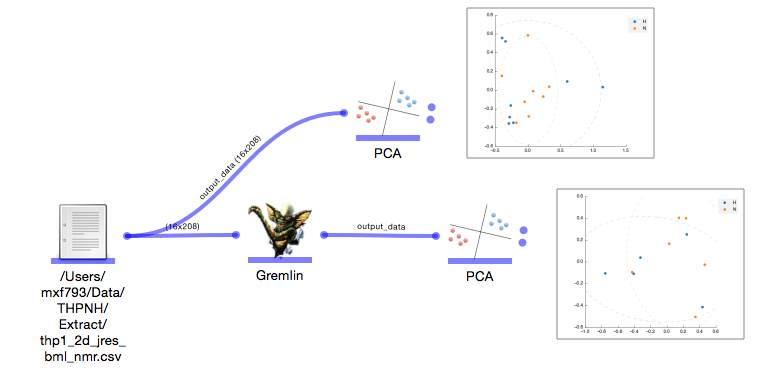
QGraphics Framework in PyQt5
Vector graphic interfaces in PyQt5
The Qt Graphics View framework is a scene-based vector graphics API. Using this you can create dynamic interactive interfaces for anything from vector graphics tools, data analysis workflow designers to simple 2D games. The Graphics View Framework allows you to develop fast & efficient scenes, containing millions of items, each with their own distinct graphic features and behaviors.
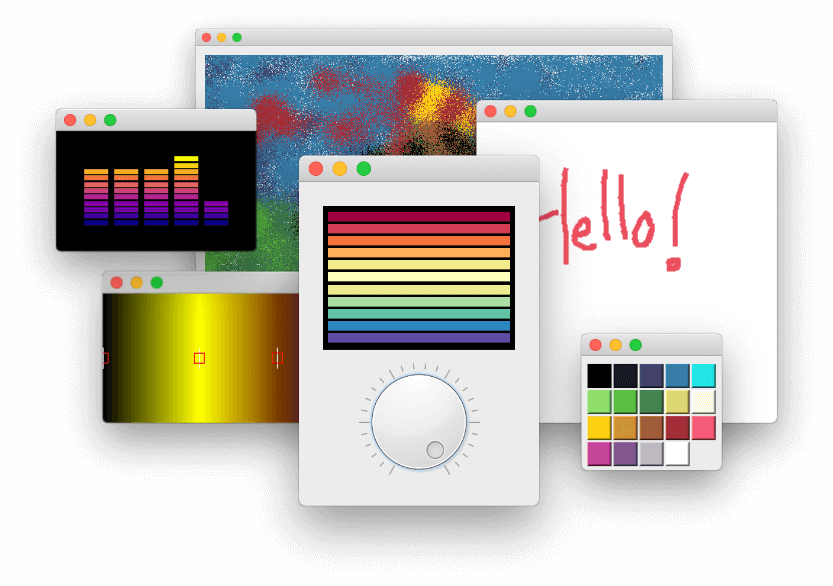
PyQt5 Custom Widgets
Designing your own custom widgets in PyQt
Widgets in Qt are built on bitmap graphics — drawing pixels on a rectangular canvas to
construct the "widget". To be able to create your own custom widgets you first need to understand
how the QPainter
system works and what you can do with it. In this PyQt5 tutorial we'll go
from basic bitmap graphics to our own entirely custom widget.
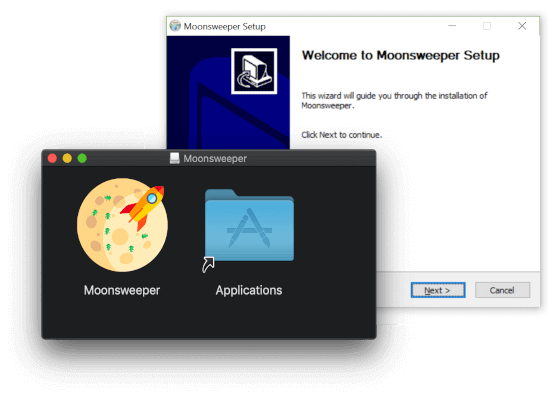
Packaging and Distributing PyQt5 Applications
Sharing your awesome applications with other people
There comes a point in any app's development where it needs to leave home — half the fun in writing software is being able to share it with other people. Packaging Python GUI apps can be a little tricky, but in this PyQt5 tutorial we'll cover how to package up your apps to share, whether commercially or just for fun.
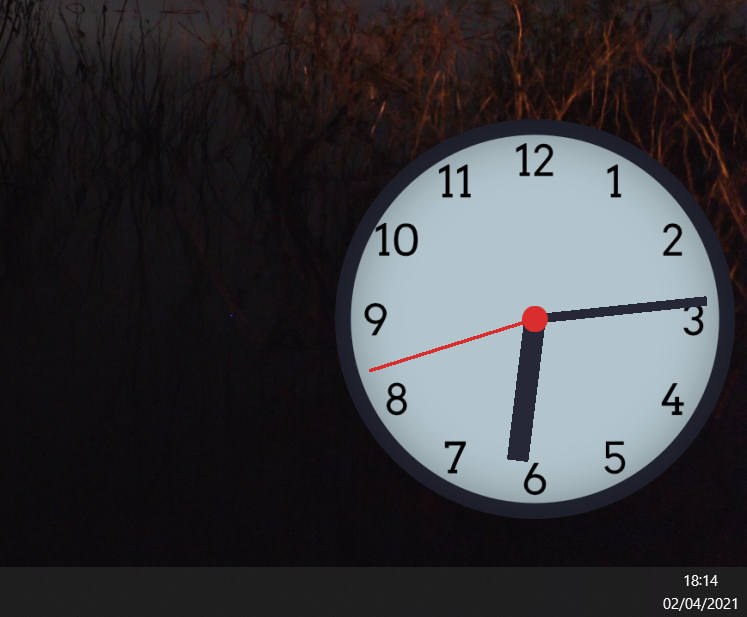
QtQuick & QML in PyQt5
Building modern PyQt5 GUIs with QtQuick & QML
Qt Quick is Qt's declarative UI design system, using the Qt Modeling Language (QML) to define custom user interfaces. Originally developed for use in mobile applications, it offers dynamic graphical elements and fluid transitions and effects allowing you to replicate the kinds of UIs you find on mobile devices. Qt Quick is supported on all desktop platforms too and is a great choice for building desktop widgets or other interactive tools. Qt Quick is also a great choice for developing UIs for hardware and microcontrollers with PyQt5.